Fundamentals of C#
Variables: Variables are used to store values of a particular datatype.
Syntax:
access_modifier datatype VariableName
Constants: Constants are used to maintain a value throughout a program.
Syntax:
const datatype ConstantName = value;
Fundamental Types Of C#
C# divides data types into two fundamental categories
Value Types
Represent actual data
Just hold a value in memory
Are stored in a stack
int, char, structures
Reference Types
Signify a pointer or a reference to the data
Contains the address of the object in the heap
= null means that no object has been referenced
classes, interfaces, arrays, strings
Data types in C#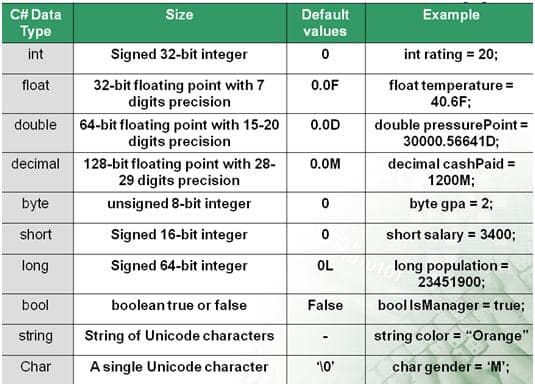
Boxing & Unboxing
Boxing is conversion of a value type into a reference type
Unboxing is the conversion of a reference type into a value type
With Boxing and unboxing one can link between value-types and reference-types by allowing any value of a value-type to be converted to and from type Object.
- Operators in C#Arithmetic
- Comparison
- Ternary (Conditional)
- Assignment
- Logical
Iteration Constructs
To perform a set of instructions for a certain number of times or while a specific condition is true.
Types of iteration constructs –
The While Loop
The Do Loop
The For Loop
The foreach Loop
Arrays
A group of values of similar data type
Belong to reference type and hence arrays are stored on the heap
The initialization or assigning of values to the array elements can be done when it is declared or at a later stage in the program.
Syntax:
DataType [number of elements] ArrayName;
exa : int[4] array1;
Structures
Custom data Types
Can have methods defined within them
Cannot implement inheritance
Represent Value types
exa:
struct student
{
public int stud_id;
public string stud_name;
public float stud_marks;
public void show_details()
{
//display student details
}
Enumerators
Enums (abbreviation for Enumerators) are a set of named numeric constants.
Used to define a data type that can have a specific set of values
Enumerators are defined using the keyword enum.
exa:
public enum WeekDays
{
Monday=1,
Tuesday=2,
Wednesday=3,
Thursday=4,
Friday=5
}
Your method of describing everything in this article is
really nice, all be capable of without difficulty understand it, Thanks a
lot.